Project 1: Adding Numbers
Your 1st homework project is to create a program that adds up a series of numbers. When you've finished your project it will look something like the image below.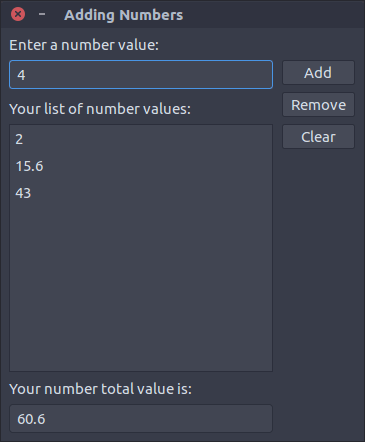
Instructions
To complete this project follow these steps.You will need to write code for the event handlers to complete this homework project.
1. Create a new folder and project in your homework folder called addnumbers.
2. Using the component list add 3 labels, 3 buttons, 2 edits, and 1 listbox to your main window.
3. Arrange them like in the layout in the image above using the appropriate caption text and positions.
4. Change the form name to AddNumbersForm, the edits to InputEdit and TotalEdit, and the listbox to NumberListBox.
5. Add click event handlers to the 3 buttons.
For the AddButton handler you will need to use the functions StrToFloatDef and FloatToStr to take a value from the top edit control and add it to the listbox. Here is an example of how to add a number to a listbox assuming N is a variable holding a number.
var N: Double; begin N := StrToFloatDef(InputEdit.Text, 0) NumberListBox.Items.Add(FloatToStr(N)); UpdateTotals; end;As adding 0 to running total does not alter a sum total, you should place an if statement in the code above. The if statement should prevent adding the number 0 to the NumberListBox.
After a number has been added or removed from NumberListBox you'll need to update the total value at the bottom of you window. To compute a total you can use code like this below.
procedure TAddNumbersForm.UpdateTotals; var N: Double; I: Integer; begin N := 0; for I := 0 to NumberListBox.Items.Count - 1 do N := N + StrToFloat(NumberListBox.Items[I]); TotalEdit.Text := FloatToStr(N); end;If you use the code above you'll need to make sure you form class has the UpdateTotals in its interface section. You can ensure this is the case by placing your editing cursor inside the letters UpdateTotals and pressing Ctrl + Shift + C to invoke the class completion tool within the IDE.
Finish the project by adding logic to remove and clear items from the NumberListBox. In your remove and clear event handlers be sure to call the UpdateTotals method to compute the new total.
If you are unsure of how to accomplish some tasks within this homework project you way want to refer to our prior recent projects "adding numbers" and "managing a list".