Project 2: Dual List Boxes
Your 2nd homework project is to create a program which allows users to select items from one list and add them to another. When you've finished your project it will look something like the image below.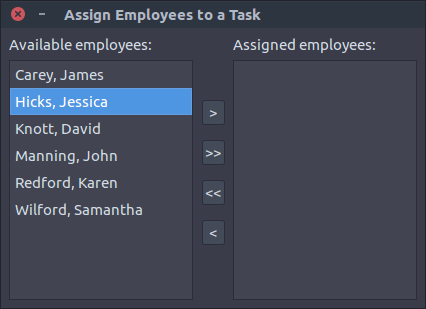
Instructions
To complete this project follow these steps.You will need to write code for the event handlers to complete this homework project.
1. Create a new folder and project in your homework folder called listselect.
2. Using the component list add 4 buttons, 2 listboxes, and 2 labels to your main window.
3. Arrange them like in the layout in the image above using the appropriate caption text and positions.
4. Change the form name to ListSelectForm, the listbox on the left to LeftBox and the one on the right to RightBox. The buttons should be named AddButton, AddAllButton, RemoveAllButton, and RemoveButton from top to bottom.
5. Enabled multiselect for both listboxes.
6. Add the following names to the LeftBox.Items property.
Carey, James
Hicks, Jessica
Knott, David
Manning, John
Redford, Karen
Wilford, Samantha
7. Add click event handlers to the 4 buttons.
The AddButton handler should remove any selected items from the LeftBox and add them to the RightBox. Here is a bit of example code to help you get started.
for I := LeftBox.Items.Count - 1 downto 0 do if LeftBox.Selected[I] then begin RightBox.Items.Add(LeftBox.Items[I]); LeftBox.Items.Delete(I); end;After items have been moved from on list to another you can sort them alphabetically using the following procedure.
procedure SortItems(Items: TStrings); var Temp: TStringList; begin Temp := TStringList.Create; Temp.Assign(Items); Temp.Sorted := True; Items.Clear; Items.Assign(Temp); Temp.Free; end;Which can be use use to sort either listbox like so:
SortItems(RightBox.Items);Add an event handler to AddAllButton to move all items (even if they aren't selected) from LeftBox to RightBox. Add code to ensure RightBox items are sorted afterwards.
Add an event handler to RemoveAllButton to move all items (even if they aren't selected) from RightBox back to LeftBox. Add code to ensure LeftBox items are sorted afterwards.
Add an event handler to RemoveButton to move only the selected items from RightBox back to LeftBox. Add code to ensure LeftBox items are sorted afterwards.
If you are unsure of how to accomplish some tasks within this homework project you may want to refer to our prior recent projects which made use of listbox controls.