Project 3: Book Reader
Your 3rd homework project is to create book reader program. When you've finished your project it will look something like the image below.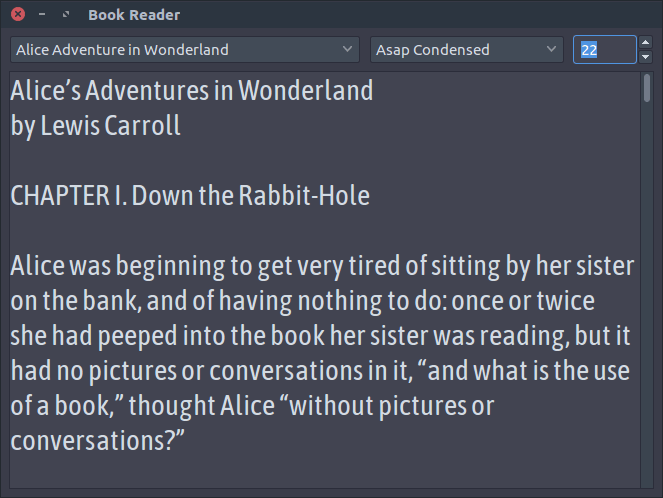
Instructions
To complete this project follow these steps.You will need to write code for the event handlers to complete this homework project.
1. Create a new folder and project in your homework folder called books.
2. Using the component list add 2 comboboxes, 1 edit, 1 updown, and 1 memo to your main window.
3. Arrange them like in the layout in the image above using the appropriate caption text and positions.
4. Change the form name to BooksForm, the first and second comboboxes to FileBox and FontBox. The edit and updown should be named SizeEdit and SizeUpDown respectively. The memo should be named ContentMemo.
5. Change the combobox style for both comboboxes to csDropDownList. Set the associate of the updown to SizeEdit. Remove the text from the memo and edit controls. Set the min and max values of the updown to 4 and 144
6. Add theses event handlers: oncreate to the form, onchange to the comboboxes, onkeypress to the edit, onchangingex to the updown.
7. Copy the book-data folder and its contents to you project folder.
Per setup step 7 above, the books for this project are should be placed in a folder called 'book-data'. Here is a bit code you can use to find the file names of the books included in this project.
const BookData = 'book-data/'; procedure FindBooks(Books: TStrings); var Search: TSearchRec; Files: TStringList; begin Files := TStringList.Create; try if FindFirst(BookData + '*', faAnyFile and not (faDirectory), Search) = 0 then repeat Files.Add(Search.Name); until FindNext(Search) <> 0; FindClose(Search); Files.Sorted := True; finally Books.Assign(Files); Files.Free; end; end;Use the FindBooks procedure in the form's oncreate event handler to populate the FileBox items. In that same event handler populate the FontBox items using Screen.Fonts on the right hand side of an assignment statement.
Write code in the FileBox onchange event handler to load the currently select file into the ContentMemo. The name of the current file can built using the expression BookData + FileBox.Text.
Write code in the FontBox onchange event handler to alter the Font.Name of ContentMemo.
Write code in the SizeEdit onkeypress event handler to detect when enter is pressed and alter the Font.Size of ContentMemo. Make sure the size is no less and no more than the Min and Max values of SizeUpDown.
Write code in the SizeUpDown onchangingex event handler to alter the Font.Size of ContentMemo using the new value.
If you are unsure of how to accomplish some tasks within this homework project you may want to refer to our prior recent projects or search for help using the documentation.