OpenGL Fragment Shader Problem
I am testing an example shader program that exhibits a peculiar problem when dealing with attributes. The problem seems to be that unless I make use of an attribute passed to the vertex shader, then attributes that follow it are moved to a different location. In the example vertex shader listed at the end of this page, if I do not reference an unused "texcoord" attribute the "normal" attribute becomes corrupted.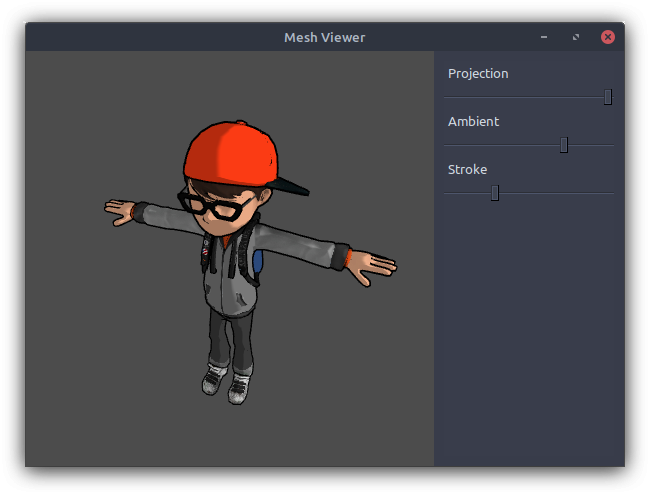
My application is using two shader programs referencing the same VBO. The second program that draws with a texture uses all three attributes, but the first one, which draws the black character outline, only needs the first and third attributes. I thought to myself, meh I can just use the same VBO and ignore the texture coordinates in the my outline shader, but if I do that the normal attribute becomes corrupted. If I add an unnecessary "if" that references the unused texcoord attribute, then everything works.
My question is why does my GLSL shader and program compiler do this and is this a standard thing or a quirk?
#version 130 // This shader produces a black outline, and texcoord isn't really needed attribute vec3 vertex; attribute vec2 texcoord; attribute vec3 normal; uniform mat4 view; uniform mat4 model; uniform mat4 perspective; uniform mat4 orthographic; uniform float blend; uniform float stroke; void main() { vec3 v = vertex; // When the "if" line below is removed this shader produces incorrect results. Why? if (texcoord.x > 0.0 || texcoord.x > 0.0) // This line is always true v = v + normal * stroke * 3.0; vec4 a = perspective * view * model * vec4(v.x, v.y, v.z, 1.0); vec4 b = orthographic * view * model * vec4(v.x, v.y, v.z, 1.0); gl_Position = (a * blend) + (b * (1.0 - blend)); }